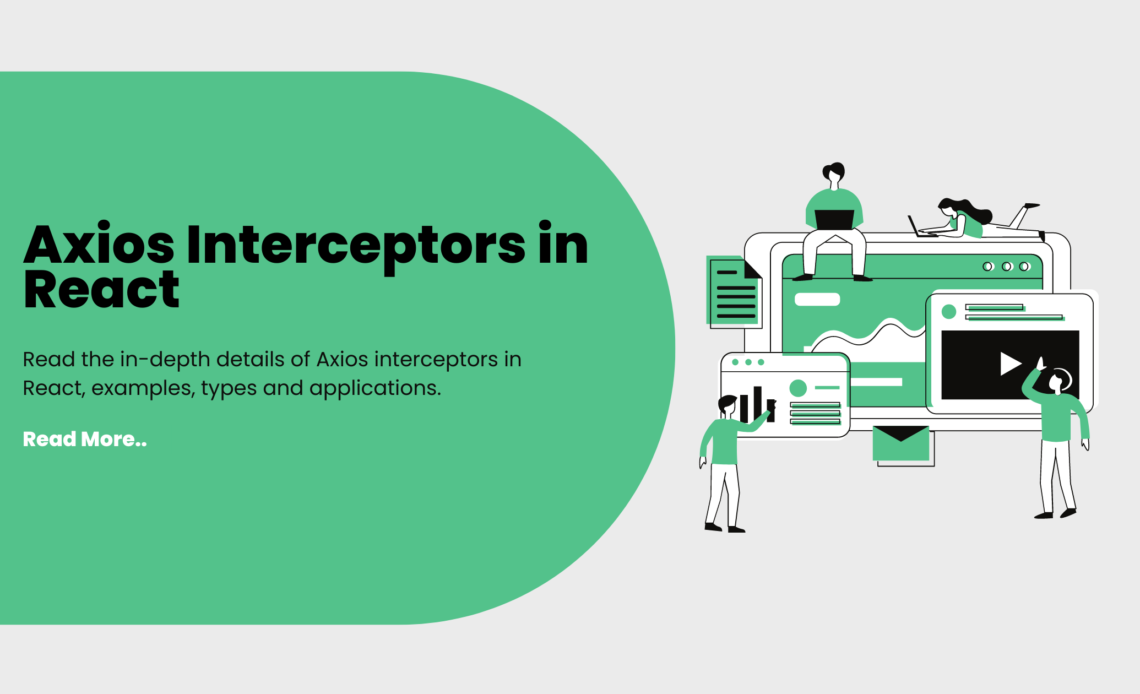
React is a view library that does much of the work for you regarding updates and changes in the DOM. It makes managing your state and taking care of your UI easy. React is one of the popular front-end frameworks.
Every dynamic UI needs to interact with the backend, and the most popular way of doing this is using HTTP calls. Most of the time, a particular part of a code could be a header or specific operation that you need to perform to an HTTP call before dispatching the request or receiving the response. Product managers can take their project’s interactivity to the next level by correctly understanding how to use HTTP calls. In Angular, it has been added as a feature with a module. To get the same functionality in React, we use an interceptor. Here we are discussing Axios Interceptors with React, its types, examples and applications.
Every time a user requests or receives a response, default configurations (or “interceptors”) run in the background to ensure everything is functioning correctly. These configurations are called “axios interceptors.” It is good practice to check the response status code for every response received to ensure no errors have occurred.
Interceptors are like middleware for Axios or Express. They allow you to run some code before your request is sent (and after receiving the response). Given are the two different types of Axios Interceptors with react.
Two Types of Interceptors
There are mainly two interceptors because an API call comprises two parts: a request and a response. The request is sent first, and then the response is received. The two halves of an API call are separate, like a checkpoint. This means that there are different interceptors for the request and the response.
Request interceptor – The request interceptor gives you the ability to write or execute a piece of code before the request is sent. This is useful for times when you want to make sure that all of the data is in the correct format before it gets sent off.
Response interceptor – It allows you to have control over when your code is executed in relation to when a response is received.
Axios Interceptors with React Example
index.jsx
index.jsx
import React from ‘react’;
import App from ‘./App’;
import ReactDOM from ‘react-dom’;
import axios from ‘axios’;
// For GET requests
axios.interceptors.request.use(
(req) => {
// Add configurations here
return req;
},
(err) => {
return Promise.reject(err);
}
);
// For POST requests
axios.interceptors.response.use(
(res) => {
// Add configurations here
if (res.status === 201) {
console.log(‘Posted Successfully’);
}
return res;
},
(err) => {
return Promise.reject(err);
}
);
ReactDOM.render(
<React.StrictMode>
<App />
</React.StrictMode>,
document.getElementById(‘root’)
);
App.jsx
import React, { useEffect, useState } from ‘react’;
import ‘./App.css’;
const App = () => {
const [data, setData] = useState(null);
const [val, setVal] = useState(”);
const [fetchData, setFetch] = useState(false);
useEffect(() => {
if (fetchData) {
const payload = {
method: ‘POST’,
body: JSON.stringify({ title: val }),
};
axios.post(‘https://jsonplaceholder.typicode.com/posts’, payload)
.then((res) => setData(res.data.id));
}
}, [fetchData]);
return (
<>
{data && <h1>Your data is saved with Id: {data}</h1>}
<input
placeholder=”Title of Post”
value={val}
onChange={(e) => setVal(e.target.value)}
/>
<button onClick={() => setFetch(true)}>Save Data</button>
);
};
export default App;
Steps to create Axios Request & Response Interceptors with React
Here are the 4 main steps to create the Axios request and response interceptors with React.
- Create a new Axios instance in react with a custom config
- Create request handler, response handler & error handlers
- Configure/make use of request interceptor & response interceptors from Axios
- Export the newly created Axios instance to be used in different locations
Applications of Request interceptor
If you want to check your credentials before making a request, you can check at the interceptor level. This way, you can ensure that your credentials are valid before making an API call.
If you need to attach a token to every request made, you can create an interceptor that will do this automatically for every request. This will save you from duplicating the token addition logic at every Axios call.
Applications of Response Interceptor
Assuming you receive a response from the API, you can deduce that the user is logged in. So, in the response interceptor, you can initialize a class that handles the user logged-in state and update it accordingly on the response object you received. This will help keep your project organized and ensure the user’s login state is updated correctly.